What is the queueable class in Apex Salesforce?
Imagine you have a long-running report that needs to analyze a ton of data. Running this synchronously could slow down your Salesforce instance and make it unresponsive. This is where Queueable Apex comes in. You can schedule this report to run asynchronously using a Queueable Apex class, and it will execute in the background without impacting your user experience.
Queueable Apex classes are super useful because they allow you to:
Run long-running operations: Tasks like batch processing, sending emails to many recipients, or complex calculations can be handled efficiently.
Improve user experience: By offloading these tasks, you prevent your users from experiencing slowdowns or delays.
Avoid governor limits: Asynchronous operations are less prone to hitting governor limits, which can occur with synchronous processing.
Here’s how Queueable Apex works:
1. Create a class: You create a class that implements the Queueable interface.
2. Define the execute method: This method contains the code that will be executed asynchronously.
3. Queue the job: You use the System.enqueue() method to add the job to the queue.
4. Asynchronous execution: Salesforce handles the asynchronous execution of the job in the background.
To understand how Queueable Apex works, let’s imagine you want to send a personalized email to each customer in your database. You could create a Queueable Apex class with a method that iterates through the customer list and sends the email to each customer. You would then queue this job using the System.enqueue() method. Salesforce would then handle the asynchronous execution of the job, sending the emails one by one in the background without affecting your users’ experience.
What is the difference between batch apex and Queueable apex in Salesforce?
Think of Batch Apex like a powerful engine designed to handle large amounts of data. If you need to process thousands or even millions of records, Batch Apex is your go-to. It breaks your processing into manageable chunks called “batches,” ensuring your system doesn’t get bogged down.
Queueable Apex is more like a nimble, efficient worker. It’s perfect for smaller jobs or tasks that require quick processing. Queueable Apex excels at handling shorter-lived tasks, like sending emails or updating a few records.
Here’s a simple way to remember the difference:
Batch Apex: For processing *large* amounts of data. Think big!
Queueable Apex: For handling *smaller* tasks. Think quick!
Batch Apex has a slightly higher limit: you can have up to five tasks running simultaneously, while Queueable Apex allows you to run up to 100 at once. This means that Queueable Apex can handle a higher volume of smaller jobs, making it a good choice for scenarios where you need many tasks to complete quickly.
But don’t get confused! Both Batch Apex and Queueable Apex are important parts of the asynchronous processing toolbox in Salesforce. They’re there to help you write cleaner, more efficient code, and to ensure that your users have a smooth experience even when you’re working with large volumes of data.
How many numbers of jobs can I queue using system enqueueJob() at a time?
Let’s dive a little deeper into how this works. Apex transactions are like little bundles of code that run together. When you use System.enqueueJob, you’re essentially adding a job to a “to-do list” for the system to handle later.
Think of it like a real-world to-do list. You might have a bunch of tasks you need to get done, but you only have so much time to work on them at once. You might decide to tackle a few tasks right away, and then add the rest to your to-do list to work on later.
That’s exactly what’s happening with System.enqueueJob. You can add a certain number of jobs to the queue, and Salesforce will process them in the background when it has the resources available.
The 50-job limit is in place to help prevent overloading the system. If you were able to add an unlimited number of jobs, it could lead to performance issues and slow down other processes. The 50-job limit helps ensure that Salesforce can handle all of the tasks that are being added to the queue efficiently.
How to call queueable?
Now, the method within your class that implements the Queueable interface needs to be declared as either public or global. This ensures that other parts of your Salesforce org can access and use your code. If you plan on making calls to external systems from your Queueable Apex, you’ll need to add the `Database.AllowsCallouts` annotation to your method. This tells Salesforce that it’s okay for your code to make those calls.
Here’s how to think about this:
– Queueable: The key to making your Apex code run in the background, away from the user’s interaction.
– Public or Global: You want your Queueable code to be accessible to other parts of Salesforce.
– Database.AllowsCallouts: You need this if your code needs to communicate with external systems.
Calling a Queueable Apex
Okay, you’ve got your Queueable Apex class all set up. Now, how do you actually make it run? That’s where the System.enqueueJob() method comes in. You pass your Queueable class to this method, and it kicks off the process of running your code in the background.
Here’s an example:
“`java
// Our Queueable class
public class MyQueueable implements Database.Queueable {
public void execute(Database.QueueableContext context) {
// Your code here to run asynchronously
}
}
// Calling the Queueable class
MyQueueable job = new MyQueueable();
System.enqueueJob(job);
“`
In this example, the `MyQueueable` class implements the `Database.Queueable` interface. When you call `System.enqueueJob(job)`, Salesforce takes the `MyQueueable` class, puts it in the queue, and then runs the code in the `execute` method asynchronously.
Benefits of using Queueable Apex:
– Improved Performance: Queueable Apex allows you to run long-running processes in the background, freeing up the user interface for immediate interactions.
– Scalability: Queueable Apex helps you handle large volumes of data and complex operations without impacting the performance of your Salesforce org.
– Reliability: By running asynchronously, Queueable Apex ensures that tasks are completed even if a user’s session times out or the browser closes.
Can we call Queueable apex from batch?
Queueable Apex lets you chain up to 50 jobs, while in the Developer Edition, you’re limited to 5 jobs. This is great for when you need to break down a large task into smaller, manageable chunks. On the other hand, Batch Apex allows up to 5 concurrent jobs to run at the same time, which is perfect for parallel processing.
Let’s dive a bit deeper into why you might want to call Queueable Apex from your Batch Apex class. Imagine you’re processing a huge dataset, and each record needs some external data. You can use Batch Apex to break down the dataset into manageable chunks, but then you might want to use Queueable Apex to handle the external data retrieval for each chunk. This way, you avoid long-running transactions and potential timeouts, ensuring your code executes smoothly. You can also use Queueable Apex to perform actions after a Batch Apex job is complete. For example, if your Batch Apex job updates a lot of records, you could use a Queueable Apex job to send out notifications to affected users.
The key takeaway is that Queueable Apex can be a powerful tool within your Batch Apex classes, allowing you to design complex, robust, and efficient solutions.
What is the difference between future method and Queueable apex?
Future methods allow you to execute code asynchronously in a single transaction. This means that the code runs in the background, independent of the user’s current request. However, you’re limited to one asynchronous operation per future method call.
Queueable Apex, on the other hand, allows you to break down large amounts of data into smaller chunks and process them in multiple chained jobs. This is extremely valuable for scenarios where you need to handle a significant volume of data or need more flexibility in scheduling and managing your asynchronous operations.
Think of it this way:
* Imagine you have a big box of toys to sort. With a future method, you can only sort one toy at a time, even though you have a box full of them.
* With Queueable Apex, you can grab handfuls of toys, sort them, and then move on to the next handful, allowing you to tackle the entire box much faster.
Here’s a closer look at the differences:
| Feature | Future Method | Queueable Apex |
|—|—|—|
| Number of Asynchronous Operations | One per call | Multiple, chained jobs possible |
| Data Handling | Not ideal for large datasets | Efficient for large datasets, splitting them into manageable chunks |
| Job Scheduling | Limited control | More flexible, allowing for rescheduling even if limits are reached |
| Limits | Can be impacted by limits | Can handle large data sets with better limit management |
Queueable Apex has a key advantage: it offers Finalizers. Finalizers are special methods that execute after the completion of a Queueable job. They provide a mechanism to handle potential issues like limit exceptions or other errors that might occur during the job execution. This allows you to:
Reschedule the job: If your job hits a limit, the Finalizer can reschedule it, ensuring that the job eventually completes successfully.
Handle errors gracefully: Finalizers can capture errors, log them, or trigger other actions to ensure that problems are addressed.
In essence, Queueable Apex provides a more robust and scalable approach to asynchronous operations, especially for tasks involving large data volumes or situations requiring error handling and rescheduling capabilities.
See more here: What Is The Difference Between Batch Apex And Queueable Apex In Salesforce? | Queueable Apex Class In Salesforce
What is the difference between future and queueable apex in Salesforce?
Both Future and Queueable allow you to run long-running processes asynchronously in the background, preventing your users from experiencing performance issues. However, they have distinct characteristics and use cases.
Future Apex is simpler, using the @future annotation to mark a method as asynchronous. It’s like setting a timer for a task to execute later. This is a great option for short-lived, isolated processes.
Queueable Apex offers more flexibility and control. It involves implementing the Queueable interface, which allows you to create more complex, multi-step operations. You can also use Queueable Apex to schedule jobs and handle errors more gracefully.
Here’s a deeper look at each approach:
Future Apex
Simplicity: Use the @future annotation to mark a method as asynchronous.
Execution: Methods marked with @future are executed in the background, freeing up the current transaction.
Limitations:
* No direct access to current user information.
* Not ideal for long-running, multi-step processes.
* Limited error handling capabilities.
Queueable Apex
Flexibility: Implement the Queueable interface to create more complex, multi-step asynchronous processes.
Execution: The execute method is executed in the background.
Features:
* Provides access to the current user.
* Allows for multi-step operations with the execute method.
* Provides robust error handling mechanisms.
Use Cases:
* Large data processing tasks.
* Complex batch operations.
* Integration with external systems.
* Scheduling and recurring jobs.
In essence, think of Future as a quick way to run a task in the background. Queueable gives you more control over longer, more complex processes and allows for error handling. You can even combine Future and Queueable if you need to execute a short-lived Future method within a Queueable job for specific tasks.
How to implement queueable apex?
Here’s a breakdown of how to build your own Queueable Apex implementation:
1. The Core Components:
You’ll need a few key players to make your Queueable Apex work its magic:
AsyncJob.cls: This class serves as a generic job object, holding onto the information about the tasks that need to be processed. It’s the backbone of your queue.
GenericCallable.cls: This class is where you define the specific methods you want to run in the background. It’s like your instruction manual for the queue.
AsyncJobService.cls: This class acts as the conductor, orchestrating the entire process by managing the queue and calling the appropriate methods defined in GenericCallable.cls.
2. Building the Foundation:
AsyncJob.cls is a simple class that stores the necessary information about a queued job. Here’s a basic structure:
“`java
public class AsyncJob {
public String jobId; // Unique identifier for the job
public String jobType; // Specifies the type of job being queued
public String jobData; // Data needed for the job execution
public Boolean isCompleted; // Flag to track completion status
// … Other attributes as needed
}
“`
3. Defining Your Tasks:
Now, let’s look at GenericCallable.cls. This is where you define the methods that will be executed in the background. The crucial part here is the execute method, which takes an instance of AsyncJob as input and performs the specific actions required for your queued task.
“`java
public class GenericCallable implements Database.Batchable
public void execute(Database.BatchableContext bc) {
// Implementation for batch process
}
public void execute(QueueableContext qc) {
// Implementation for Queueable process
}
public void execute(AsyncJob job) {
// … Perform the task based on ‘jobData’
}
}
“`
4. Orchestrating the Process:
The AsyncJobService.cls takes center stage here. It acts as the middleman between your queued jobs and the GenericCallable.cls, ensuring everything runs smoothly.
“`java
public class AsyncJobService {
public static void queueJob(AsyncJob job) {
// … Store the ‘job’ in a database or other storage
// … Schedule the ‘job’ for execution using System.enqueue()
}
public static void processJob(AsyncJob job) {
// … Retrieve the ‘GenericCallable’ instance based on ‘jobType’
// … Execute the ‘execute’ method on the retrieved ‘GenericCallable’ instance
}
}
“`
5. Bringing It All Together:
To start a queued job, you would:
* Create an instance of AsyncJob, populating it with the required data.
* Use AsyncJobService.queueJob to store and schedule the job for execution.
* AsyncJobService.processJob would then retrieve the job from storage, find the appropriate GenericCallable instance, and execute the task.
Remember, the actual implementation of the execute method within your GenericCallable.cls is where you define the specific actions you want to perform for each job type.
Queueable Apex gives you the flexibility to manage long-running operations in a controlled and efficient manner. It’s a powerful tool that can significantly enhance your Salesforce applications by freeing up resources and optimizing performance.
What is a queueable apex class?
Queueable Apex Classes are like little helpers that take care of your jobs while you get on with other tasks. They’re based on the Queueable Interface, which makes sure your code runs smoothly in the background. Here’s how it works:
* You create a class that implements the Queueable Interface.
* This class needs an execute() method, which contains the actual logic you want to run.
* When you call your Queueable Apex Class, Salesforce puts it in a queue, like a virtual waiting room.
* Salesforce then takes care of executing your job, freeing up your user’s session.
Let’s take a look at the AsyncJob.cls class, which is a great example of a Queueable Apex Class in action:
“`java
public class AsyncJob implements Queueable {
private GenericCallable toExecute;
public AsyncJob(GenericCallable toExecute) {
this.toExecute = toExecute;
}
public void execute(QueueableContext context) {
toExecute.execute();
}
}
“`
Let’s break this code down:
AsyncJob is a class that implements the Queueable Interface, making it ready to run as a background task.
* GenericCallable is a simple interface that helps you pass different types of tasks to your Queueable Apex Class.
toExecute is a variable that holds the actual job you want to run.
AsyncJob’s constructor takes a GenericCallable as input, allowing you to specify the task you want to queue.
The execute() method is where the magic happens. This is where you actually execute the code that you passed in using the toExecute variable.
So, in essence, AsyncJob acts as a wrapper for the actual task you want to perform. It takes your task, puts it in the queue, and then runs it when Salesforce is ready. This means you can keep your users happy and productive, while complex processes run smoothly in the background.
How to enqueue apex job in Salesforce?
`ID jobId = System.enqueueJob (job);`
This line of code is where the magic happens. You’re calling the `enqueueJob` method, which takes your `job` (which is an instance of a class implementing the `Queueable` interface) and adds it to the Salesforce job queue.
Think of it like adding a task to your to-do list – Salesforce takes care of running the job when it has the resources available.
The `enqueueJob` method also gives you an `ID`, called `jobId`, which is a unique identifier for your job. You can use this ID to track the progress of the job, find out if it’s finished, and even cancel it if needed.
Here’s the cool thing about Queueable Apex:
You don’t have to worry about writing code to handle the actual execution of your job. Salesforce automatically manages that for you. It works behind the scenes, picking up your job and running it when the system has the available resources. Think of it as a super-efficient, dedicated team of bots running your jobs in the background so you can focus on other things.
You can also set up `System.schedule` to run your job at a specific time or at regular intervals. This is a great way to automate tasks that need to be done on a schedule, like sending out reports or cleaning up data.
Want to learn more about the `Queueable` interface and build more complex jobs?
Here’s a quick recap:
1. Create a class that implements the `Queueable` interface.
2. Define the `execute` method inside your class. This is where you’ll put the code that you want to run as part of your job.
3. Create an instance of your class and pass it to the `enqueueJob` method.
4. Use the job ID to track the status of your job.
You’ll see that you can do a lot with Queueable Apex. It’s powerful and flexible for handling tasks that can be done asynchronously.
Now, go forth and build amazing jobs!
See more new information: countrymusicstop.com
Queueable Apex Class In Salesforce: A Comprehensive Guide
Hey there, Salesforce developers! Today we’re diving deep into the wonderful world of Queueable Apex Classes. It’s a powerful feature that lets you run your Apex code asynchronously, making your applications faster and more efficient. Let’s break it down and get you up to speed.
What are Queueable Apex Classes?
Imagine you’re building a system that sends out personalized emails to thousands of customers. If you tried to send those emails all at once, your application would likely slow to a crawl. This is where Queueable Apex steps in to save the day!
Queueable Apex allows you to define classes that can be executed in the background, meaning your application can continue to run smoothly while these tasks are completed.
Think of it like this: It’s like putting a bunch of tasks into a virtual queue, and then Salesforce’s super-efficient background worker process picks them up one by one, handling them without impacting your user’s experience.
How do Queueable Apex Classes Work?
Queueable Apex leverages Salesforce’s Asynchronous Apex Execution, which means it handles your code in the background. Here’s how it works in a nutshell:
1. You create a class that implements the `Queueable` interface.
2. You define an `execute` method within your class. This is where your actual code will go!
3. You create an instance of your Queueable class and pass it to the `System.enqueue` method.
4. Salesforce takes over, queuing your task and executing it later.
Building Your First Queueable Apex Class
Let’s get our hands dirty and create a simple Queueable class. Imagine we’re building a system to update a bunch of Account records. Here’s the code:
“`java
public class UpdateAccountBatch implements Queueable {
public void execute(QueueableContext context) {
// Update Account records here
List
for (Account account : accountsToUpdate) {
account.Industry = ‘Technology’;
update account;
}
}
}
“`
In this code:
* We declare a class `UpdateAccountBatch` that implements the `Queueable` interface.
* The `execute` method takes a `QueueableContext` as an argument, which provides information about the current execution.
* Inside the `execute` method, we retrieve accounts matching a specific criteria and update them.
Now, to run our code asynchronously, we just need a simple line:
“`java
System.enqueue(new UpdateAccountBatch());
“`
That’s it! This code line adds our `UpdateAccountBatch` to the queue, and Salesforce will handle the rest.
Benefits of Using Queueable Apex
Using Queueable Apex has several advantages:
Improved Performance: Your code runs in the background, so your application remains responsive, even when processing large amounts of data.
Increased Scalability: You can handle more requests and transactions without bogging down your system.
Reduced Resource Consumption: By running tasks asynchronously, you free up resources for other operations.
Flexibility: You can schedule your Queueable Apex tasks to run at specific times or intervals.
FAQs
Q: Can I use Queueable Apex for long-running processes?
A: Yes! You can even use Queueable Apex for tasks that take longer than the standard Apex governor limits (10 seconds).
Q: How do I handle errors in Queueable Apex?
A: You can use try-catch blocks in your `execute` method to handle any exceptions.
Q: Can I use Queueable Apex to send emails asynchronously?
A: Absolutely! Use `Messaging.sendEmail` within your `execute` method.
Q: Are there any limits on Queueable Apex?
A: Yes, there are limits on the number of queued items and the duration of background execution. Be sure to consult the Salesforce documentation for details.
Q: What are some practical use cases for Queueable Apex?
A: You can use Queueable Apex for a wide range of use cases, including:
Batch Processing: Processing large datasets, such as importing data or updating records.
Email Sending: Sending personalized emails to many customers.
Data Integration: Pulling data from external systems.
File Handling: Uploading or downloading files in the background.
Asynchronous Processes: Running any process that doesn’t need to be completed immediately.
Tips for Using Queueable Apex
Keep Your Code Short and Simple: Break down long processes into smaller, manageable chunks.
Handle Errors Gracefully: Use try-catch blocks to handle errors and log them for debugging.
Use the QueueableContext: Utilize the `QueueableContext` to access information about the current execution.
Test Thoroughly: Always test your Queueable Apex code to ensure it runs correctly.
Conclusion
Queueable Apex is a powerful tool that helps you improve the performance and scalability of your Salesforce applications. By understanding how to use Queueable Apex, you can create more efficient and robust applications. Remember to practice, experiment, and explore the full potential of this awesome feature!
Queueable Apex | Apex Developer Guide | Salesforce
Take control of your asynchronous Apex processes by using the Queueable interface. This interface enables you to add jobs to the queue and monitor them. Using the interface is an enhanced way of running your asynchronous Apex code compared to using future Salesforce Developers
Understand Queueable Apex with Example and Test
Create a Queueable Apex class that inserts the same Contact for each Account for a specific state. Write unit tests that Salesforce Bolt
Control Processes with Queueable Apex | Salesforce Trailhead
Queueable Apex allows you to submit jobs for asynchronous processing similar to future methods with the following additional benefits: Non-primitive types: Your Queueable Trailhead
Queueable Interface | Apex Reference Guide
To execute Apex as an asynchronous job, implement the Queueable interface and add the processing logic in your implementation of the execute method. To implement the Salesforce Developers
Queueable Apex in Salesforce – The Ultimate Guide
Queueable Apex, as a feature in Salesforce, introduces a more robust and flexible way to run asynchronous Apex code. It complements the existing functionality of saasguru
Generic Approach to Salesforce Queueable – Apex Hours
Summary. Technical Overview. This implementation simply consists of three parts. A generic job object, AsyncJob.cls. A callable class for class/method configuration, GenericCallable.cls. A service class for Apex Hours
Queueable Apex | Apex Developer Guide | Salesforce
Apr 8, 2020. Interviewer: What is Queueable Apex? Interviewee: It’s an extension of the Future Methods. It uses the Queueable interface which is an enhanced way of running your asynchronous… Medium
Queueable Apex in Salesforce with example – Salesforce Geek
In this blog, we are going to discuss Queueable Apex in Salesforce with example. Queueable Apex is another asynchronous type of apex. It runs in a separate Salesforce Geek
Comprehensive Guide to Queueable Apex in Salesforce
What is Queueable Apex? Developers use it as an asynchronous Apex job to offload non-time-critical tasks for processing in the background, providing improved performance innostax.com
Future Vs Queueable Apex – Apex Hours
Salesforce Developer. Future Vs Queueable Apex. Prakash Jada. Mar 17, 2023. 15 Comments. What is the difference between future method and Queueable Job in Apex? Join us to learn Apex Hours
Queueable Apex In Salesforce
Salesforce – Queueable Apex
06 Example – Queueable Apex Implementation With Test Class | Asynchronous Apex In Salesforce
Control Processes With Queueable Apex || Asynchronous Apex || Salesforce
Queueable Apex With An Example
Link to this article: queueable apex class in salesforce.
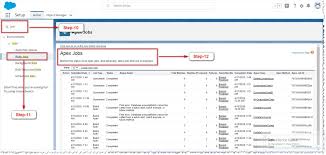
See more articles in the same category here: blog https://countrymusicstop.com/wiki