How do you define comp-3 in COBOL?
COMP-3 fields are super efficient because they store numbers in a compact way. Think of it like this: You can squeeze a lot more numbers into a smaller space. This is great for saving memory and optimizing your code.
Here’s the deal with COMP-3 fields:
Packed Decimal: Instead of using a full byte for each digit, COMP-3 packs two digits into a single byte. The only exception is the last byte, which holds the sign of the number.
Sign Field: The last byte tells you whether the number is positive or negative. Here’s the breakdown:
x’C’: This means the number is positive.
x’D’: This means the number is negative.
x’F’: This means the number is unsigned. It’s like saying, “This number is not positive or negative.”
Implied Decimal Point: You can’t see the decimal point in the field, but it’s there! The COMP-3 field definition specifies where the decimal point goes. This helps you work with numbers that have fractional parts.
Here’s a simple example:
Imagine you have a COMP-3 field defined as PIC S9(4) COMP-3. This means the field can hold a number with 4 digits, including a sign, and it has an implied decimal point between the second and third digits.
So, if the COMP-3 field contains the value x’1234C’:
* It represents the number 12.34.
* The ’12’ are stored in the first byte.
* The ’34’ are stored in the second byte.
* The ‘C’ in the third byte indicates a positive number.
Important Notes:
COMP-3 fields can be very efficient for numeric calculations, especially when you are working with large volumes of data.
* You can use the COMP-3 data type when you need to store numeric values in a compact and efficient way.
* Remember that the COMP-3 data type is specific to COBOL and may not be available in other programming languages.
How do I see the value of the field stored in comp-3 format?
COMP-3 format, also known as packed decimal, is a way to store numeric values in a more compact and efficient way compared to zoned decimal. It does this by packing two digits into each byte. This is achieved by using the high-order and low-order nibbles of each byte.
Think of it like this: you have a byte, which is like a small box with eight spaces. You can store two digits in each box, one in the top four spaces (high-order nibble) and the other in the bottom four spaces (low-order nibble). The high-order nibble of the first byte holds the most significant digit of the value, and you continue reading the digits from left to right, working your way down to the low-order nibble of the last byte.
For instance, let’s say you have a COMP-3 field that’s three bytes long. This means you can store a maximum of six digits (three bytes x two digits per byte). If the value stored is 123456, it would be represented in COMP-3 as follows:
| Byte | High-order Nibble | Low-order Nibble |
|—|—|—|
| 1 | 01 | 2 |
| 2 | 3 | 4 |
| 3 | 5 | 6 |
Seeing the value of a COMP-3 field in practice can be tricky, as it’s not immediately readable like a plain text field. You’ll need a tool that understands the COMP-3 format and can translate it for you.
If you’re working with a programming language or a tool that has built-in support for COMP-3 fields, it might automatically handle the conversion for you. For example, in COBOL, you can use the DISPLAY statement to see the value in a human-readable format.
Alternatively, if you’re working with a file or database that contains COMP-3 fields, you might need to use a dedicated tool to convert the data to a readable format. There are various tools available, both free and paid, that specialize in converting data formats, including COMP-3.
Remember, understanding COMP-3 is crucial when working with legacy systems or databases. By recognizing the format and how the digits are packed, you can ensure you’re working with the correct data and avoid any issues in your application.
Let me know if you’d like to explore specific tools or code examples to help illustrate the conversion process further. I’m happy to assist you in diving deeper into this fascinating world of data storage!
What is packed decimal in COBOL?
Packed decimal is a clever way COBOL stores decimal numbers. Think of it like a space-saving strategy. Each byte, which is 8 bits, stores two decimal digits. This means we can pack more digits into a smaller space compared to other number representations.
For example, if you have the number 123.45, a packed decimal representation would store it like this:
Byte 1: 12
Byte 2:34
Byte 3:5 (The sign is usually stored in the last half-byte.)
This method is super handy because it keeps our decimal data accurate. There’s no need for separate parts to represent the whole number and the fraction—the informat or format dictates where the decimal point goes.
Let me give you a real-world example: Imagine you’re managing a store’s inventory. You need to track prices, quantities, and other monetary values. Packed decimal helps you do this efficiently. It ensures that your calculations and data storage are precise, even if you’re working with cents or fractions of a cent!
In summary, packed decimal is a great way to handle decimal numbers in COBOL, making it perfect for financial applications and scenarios where accuracy is crucial.
How do you display negative numbers in COBOL?
When you define a numeric variable using a PIC clause in COBOL, you can control how negative numbers are displayed.
Here’s the key: The minus sign will show up as a “-” for a negative value, but you’ll see a space if the value is positive.
If you want to display a plus sign instead of a space for positive values, and still use a minus sign for negative numbers, you can use a plus sign “+” in the PIC clause.
For example, if you define a variable with PIC S9(4)V99, negative values will be displayed with a minus sign, and positive values will be displayed with a space.
Let’s say you want to display a plus sign for positive values. You would use a PIC S9(4)V99+.
Now, the variable will display a plus sign for positive values and a minus sign for negative values.
This allows for consistent formatting of your numeric data, making it easier for users to read and understand the values.
You can also customize the format further by adding other symbols like commas, periods, or dollar signs to your PIC clause. This will help to display your numeric values in a way that is both clear and visually appealing.
What is the format of comp in COBOL?
Let’s break down how this works with an example. Imagine a COMP field with a length of 5. This field can store a five-digit number, where each digit takes up four bits in a byte. For example, the number 12345 would be stored as follows:
Byte 1: 0000 0001 (representing the digit 1)
Byte 2: 0000 0010 (representing the digit 2)
Byte 3: 0000 0011 (representing the digit 3)
Byte 4: 0000 0100 (representing the digit 4)
Byte 5: 0000 0101 (representing the digit 5)
The COMP data type is useful because it:
Reduces storage space: Compared to PIC 9 fields, which store one digit per byte, COMP fields use half the storage space.
Improves performance: Calculations on COMP fields are typically faster than calculations on other data types because the digits are stored in a compact and readily accessible format.
This efficient storage and performance makes COMP a good choice for numeric variables that require frequent calculations, such as in accounting and financial applications.
What is comp 1, comp 2, and comp-3?
COMP-1 is a 4-byte data type. It represents a single-precision floating-point number, meaning it can store decimal values with a limited degree of precision. Think of it as a smaller container for storing numbers that might have a fractional part.
COMP-2 is a data type that handles double-precision floating-point numbers. This means it uses 8 bytes of memory to store numbers. The advantage here is that COMP-2 can hold larger and more precise numbers compared to COMP-1. It’s like having a bigger container for storing complex numbers.
COMP-3 is used for internal decimal values. It’s a way to represent decimal numbers internally in a computer, and the storage size depends on the precision required.
So, COMP-1, COMP-2, and COMP-3 are just different ways to store numbers in COBOL, and the choice depends on the specific needs of your program. Do you need to store very precise values? Or are you working with smaller numbers? Choosing the right data type ensures that your program works efficiently and accurately.
Now, let’s dive a bit deeper into COMP-3. Imagine you’re dealing with financial calculations and need to represent dollar amounts accurately, down to the penny. COMP-3 is perfect for this. It stores decimal values with a fixed number of decimal places, ensuring the precision you need. It’s like having a container specifically designed to handle money with cents.
One important thing to remember about COMP-3 is that it’s designed for internal use. This means that the value is stored and manipulated internally within the program but may not be directly displayed as a readable decimal value. You would typically use a separate data type, like PIC 9(x)V9(y), to display or format the decimal value for output.
Understanding these data types is crucial for any COBOL developer. By choosing the right data type, you can optimize your program for performance, accuracy, and readability.
What is the difference between comp and COMP3?
Comp is a binary format, which means data is stored in a sequence of 0s and 1s. This is a very efficient way to store data, as it takes up less space than other formats.
COMP3 on the other hand, is a packed decimal format. Packed decimal uses four bits to represent each digit, allowing you to store more data in a smaller space.
Here is a more detailed explanation of each:
Comp
Binary format: Data is stored as binary numbers.
Efficient: Takes up less storage space.
Commonly used: For storing integers and floating-point numbers.
COMP3
Packed decimal format: Each digit is stored in four bits, with leading zeros suppressed.
Space-saving: Allows you to store more data in a smaller space, especially for financial applications that often deal with decimal numbers.
Commonly used: For storing currency values, interest rates, and other financial data.
You can think of comp as being like a traditional measuring cup: It’s simple, efficient, and works well for most applications. But, COMP3 is like a measuring cup with extra markings for smaller increments, enabling you to handle more precise quantities.
Now, let’s explore the other data formats you mentioned:
Binary: This is the most basic format, where data is represented as a sequence of 0s and 1s. Think of it as the foundation for all other data formats.
Display: This format is designed for human readability. It often uses a combination of characters, numbers, and symbols to represent data. Think of it as the “pretty” format that we see on our screens.
By understanding these different data formats, you can choose the most appropriate one for your specific needs. For example, you might use comp to store a large dataset of temperatures, and COMP3 to store the balances in a bank account.
What is the storage of comp in COBOL?
Half word: This uses 2 bytes to store the integer value.
Full word: This uses 4 bytes to store the integer value.
Double word: This uses 8 bytes to store the integer value.
You might be wondering, “How do I choose which COMP storage format is right for my program?” The answer lies in the PICTURE clause. The PICTURE clause defines the format of a data item. It includes information about the number of digits, decimal positions, and other characteristics of the data item.
Here’s how the PICTURE clause impacts COMP storage:
Half word: You can use this if your integer field has up to 4 decimal digits.
Full word: This is used when the integer field has more than 4 decimal digits and up to 9 decimal digits.
Double word: You’ll use this for integer fields that have more than 9 decimal digits.
Think of it this way: The PICTURE clause tells you how many digits you need to represent your integer. Based on that, COBOL chooses the most efficient COMP storage format to store your value. This keeps your program running smoothly and using memory efficiently.
How many bytes is COMP3?
Let’s dive a bit deeper into how COMP3 works and how it saves space. In essence, COMP3 represents each decimal digit as a 4-bit value. Since a byte consists of 8 bits, this means that you can fit two decimal digits within a single byte. This efficient storage is what gives COMP3 its packed decimal designation.
For example, if you wanted to store the number 1234 using COMP3, it would only require 3 bytes:
Byte 1: The first two digits, ’12’, would be stored in the first byte.
Byte 2: The next two digits, ’34’, would be stored in the second byte.
Byte 3: The sign of the number would be stored in the third byte.
This is a significant advantage over other data types that store numbers in a fixed-width format, such as integers, which often require a full byte for each digit. While this might seem like a small difference, the savings can be substantial when dealing with large datasets.
Think of it like this: imagine you have a list of thousands of phone numbers, each with 10 digits. Storing this list using a standard integer data type would consume a lot of storage space. But using COMP3, you can store the same data using just 5 bytes per phone number, making it significantly more efficient.
See more here: How Do I See The Value Of The Field Stored In Comp-3 Format? | How To Convert Comp 3 To Readable Format In Cobol
How to define a comp-3 variable in COBOL program?
For example, this code snippet demonstrates how to define a COMP-3 variable named WS-PDN to hold a five-digit decimal number:
“`COBOL
01 WS-PDN PIC 9(5) USAGE IS COMP-3.
“`
In this example, WS-PDN is a variable that holds a five-digit decimal number in COMP-3 packed format. You can assign values to a COMP-3 variable just like any other numeric variable in COBOL.
Understanding the Advantages of COMP-3
The COMP-3 format offers a way to store numeric data in a more compact and efficient way compared to standard decimal representation. It achieves this by packing two digits into each byte of memory. This means that a COMP-3 variable can store a larger numeric value using less memory space.
Here’s a breakdown of how COMP-3 packing works:
Packed Decimal: Each byte in a COMP-3 variable holds two decimal digits, with the rightmost byte containing the sign (either a “C” for positive or a “D” for negative). For example, the number 12345 would be stored as “12345C” in a COMP-3 variable.
Space Savings: Because COMP-3 compresses two digits per byte, it uses less memory than standard decimal representation. This can lead to reduced memory usage and improved performance for your COBOL programs, especially when working with large datasets.
Let’s illustrate with an example:
Imagine you need to store the value 12345. If you used a standard decimal representation, you would need five bytes to store it. However, with COMP-3, you only need three bytes (two bytes for the digits and one byte for the sign).
This efficiency is especially valuable in situations where you’re dealing with large volumes of numeric data. By using COMP-3, you can save valuable memory space and potentially improve the speed of your COBOL programs.
How do I convert a COBOL file to a CSV file?
The best approach is to perform the conversion on the mainframe using COBOL and then transfer the resulting text file. This method is often the most efficient and straightforward.
Alternatively, there are tools like coboltocsv that can help you convert COBOL files to CSV using a COBOL copybook. These tools are designed specifically for this purpose and can handle various COBOL data types, including COMP-3.
Speaking of COMP-3, let’s explore this in more detail. COMP-3, also known as packed decimal, is a data format commonly used in COBOL. It’s a compact way to store numeric values, where each byte holds a digit and the sign is packed into the last nibble (half-byte). Converting COMP-3 to a readable format is a key step in the conversion process.
Here’s a breakdown of the common approaches for handling COMP-3:
1. Using COBOL:
– You can use COBOL to convert COMP-3 data to a standard numeric format. This involves moving the COMP-3 data to a numeric variable of the desired format (e.g., PIC 9(x)V99 for decimal values). Then, you can write this data to a file in a format suitable for CSV (e.g., using the WRITE statement).
2. External Tools:
– There are tools and libraries available that can convert COMP-3 data to standard numeric formats. These tools can often be integrated into your existing conversion process, simplifying the conversion steps.
Remember, converting COBOL files to CSV is a common task for data migration and integration projects. By understanding the different methods and tools available, you can choose the approach that best suits your needs and ensures a smooth and accurate conversion.
What if U get 000004040404 on comp-3 variable?
Let’s dive deeper into COMP-3 variables and understand why you might see this “blank” value.
Understanding COMP-3 Variables
COMP-3 variables are a data type commonly used in legacy systems like COBOL. They store numeric data in a compressed format, where each byte represents two decimal digits. This compression technique saves storage space and improves processing efficiency.
The “Blank” Value
In COMP-3 variables, the value 000004040404 is often used to represent a missing or empty value. This is a convention that helps differentiate between a truly zero value and a value that has not been assigned. When you see this value, it signifies that the variable has not been populated with any data.
Common Scenarios and Troubleshooting
File Input: When reading data from a file, you might encounter this value if the data field is empty or contains an invalid numeric value. This could be due to errors during file creation or data entry.
Data Conversion: If you’re converting data from one data type to another, improper conversion logic can lead to the 000004040404 value.
Initialization: If a COMP-3 variable is not explicitly initialized, it might hold this value until assigned a valid numeric value.
Debugging Tips
Data Validation: Validate your input data to ensure it conforms to the expected format and range for the COMP-3 variable.
Data Conversion: Carefully review your data conversion logic to prevent accidental conversions to invalid values.
Initialization: Always initialize your COMP-3 variables before using them to prevent unexpected “blank” values.
By understanding the behavior of COMP-3 variables and the meaning of the 000004040404 value, you can more effectively troubleshoot and handle data processing in legacy systems. Remember to always check the documentation of your specific system or language for detailed information on the COMP-3 data type.
How many digits are stored in a comp-3 field?
Imagine you have a COMP-3 field declared as PIC S9(5). This field can store a number ranging from -99999 to +99999. You might think it would take 5 bytes of storage, one for each digit. However, COMP-3 fields use a clever trick to save space.
They use only 3 bytes to store those 5 digits! This is because 2.5 bytes are dedicated to holding the digits themselves, while the remaining 0.5 bytes are reserved for the sign (positive or negative).
Here’s how the formula works to calculate the memory usage of a COMP-3 field with *n* digits:
Memory Usage = (n * 0.5) + 0.5
Let’s break down this formula:
n: Represents the number of digits in the PIC declaration.
0.5: Each digit requires half a byte (0.5 bytes) of storage.
0.5: An additional half byte is needed to store the sign.
For example, if you have a COMP-3 field with PIC S9(8), which can hold a number from -99999999 to +99999999, the memory usage would be calculated as follows:
Memory Usage = (8 * 0.5) + 0.5 = 4.5 bytes
Since memory is allocated in whole bytes, the actual storage used would be 5 bytes.
Remember that COMP-3 fields are designed to efficiently store numeric data in a packed format. By cleverly compressing digits and sign information, they minimize storage requirements without compromising the range of values they can hold. This makes them an efficient choice for storing large quantities of numeric data in your COBOL programs.
See more new information: countrymusicstop.com
How To Convert Comp-3 To Readable Format In Cobol
You see, COMP-3 is a data format that stores numbers in a packed decimal representation. It’s a space-saving technique, but it makes those numbers a bit tough to read directly. You’ll need to unpack them to make sense of them.
Here’s a breakdown of the process, step by step.
1. Understanding COMP-3
* First off, let’s get a grasp of COMP-3. It’s all about how data is stored. In COMP-3, each digit of a number is packed into a single byte. Think of it as a kind of compression for your numbers.
* Each byte in COMP-3 contains two nibbles. A nibble is just half a byte, so four bits. The most significant nibble (the left side) is used for the sign, and the least significant nibble (the right side) holds the digit value.
* For example, the number 123 would be stored as follows:
* 1: 0100 0001 (sign is positive, so the leftmost nibble is set to 0100, and the digit is 1)
* 2: 0100 0010
* 3: 0100 0011
2. The Conversion Process
* Now, to convert this COMP-3 data to something readable, we need to extract those digits. We’ll accomplish this using a COBOL program with a few key instructions.
3. The COBOL Code
“`cobol
IDENTIFICATION DIVISION.
PROGRAM-ID. CONVERT-COMP3.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-COMP3-DATA PIC S9(5) COMP-3.
01 WS-READABLE-DATA PIC X(10).
01 WS-TEMP PIC X.
PROCEDURE DIVISION.
MOVE 12345 TO WS-COMP3-DATA.
MOVE WS-COMP3-DATA TO WS-READABLE-DATA.
MOVE WS-READABLE-DATA(1:1) TO WS-TEMP.
IF WS-TEMP = X’F0′
MOVE ‘ ‘ TO WS-READABLE-DATA(1:1)
END-IF.
MOVE WS-READABLE-DATA(2:1) TO WS-TEMP.
IF WS-TEMP = X’F0’
MOVE ‘ ‘ TO WS-READABLE-DATA(2:1)
END-IF.
MOVE WS-READABLE-DATA(3:1) TO WS-TEMP.
IF WS-TEMP = X’F0’
MOVE ‘ ‘ TO WS-READABLE-DATA(3:1)
END-IF.
MOVE WS-READABLE-DATA(4:1) TO WS-TEMP.
IF WS-TEMP = X’F0’
MOVE ‘ ‘ TO WS-READABLE-DATA(4:1)
END-IF.
MOVE WS-READABLE-DATA(5:1) TO WS-TEMP.
IF WS-TEMP = X’F0’
MOVE ‘ ‘ TO WS-READABLE-DATA(5:1)
END-IF.
DISPLAY ‘Readable data: ‘ WS-READABLE-DATA.
STOP RUN.
“`
4. Explanation
1. Declaration: In this code, WS-COMP3-DATA is declared as a COMP-3 field that can hold a signed five-digit number. WS-READABLE-DATA is declared as a character field to store the converted value. And WS-TEMP is a temporary character field used for temporary storage.
2. Moving the Data: The line `MOVE 12345 TO WS-COMP3-DATA` sets the value of WS-COMP3-DATA to 12345.
3. Conversion: The heart of the conversion happens here. The line `MOVE WS-COMP3-DATA TO WS-READABLE-DATA` moves the COMP-3 value to the character field WS-READABLE-DATA. This operation implicitly unpacks the digits from the COMP-3 data.
4. Handling Sign: The next section uses a series of conditional statements (`IF WS-TEMP = X’F0′ … END-IF`) to examine each nibble in WS-READABLE-DATA. If a nibble is X’F0’, it signifies a leading zero. If it’s not, it signifies a digit. We need to clean up these leading zeroes for a readable output.
5. Displaying the Result: Finally, the line `DISPLAY ‘Readable data: ‘ WS-READABLE-DATA` displays the converted value.
5. Beyond the Code
* This code demonstrates a basic conversion process. You might have to adapt it based on the specifics of your data, like the length and sign of your COMP-3 value.
* It’s essential to understand the principles of COMP-3 storage and the conversion process. You might encounter different techniques or variations depending on your compiler or environment.
6. Important Points
* Data Types: Remember to match the data type of your COMP-3 variable and the character field you’re using for conversion.
* Sign Handling: Be aware of how signs are represented in your COMP-3 data. This information is crucial for handling negative values correctly.
* Padding: Leading zeroes, also known as padding, are common in COMP-3 fields. Make sure you handle these zeros effectively for a readable result.
7. Advantages of Using COMP-3
* Space Savings: COMP-3 offers a compact way to store numeric data. This can be beneficial in cases where storage space is limited.
* Efficiency:COMP-3 can be processed faster than other formats, such as PIC 9 or PIC X.
8. Disadvantages of Using COMP-3
* Readability: As we’ve seen, COMP-3 data isn’t very readable.
* Conversion Required: You’ll need to write code to convert COMP-3 data to a readable format for display or other uses.
* Portability: There can be differences in how COMP-3 is handled across different COBOL compilers, which can lead to portability issues.
9. Choosing the Right Format
* If you prioritize space savings and processing efficiency, COMP-3 might be a suitable choice.
* But if you need easily readable data, especially for human interaction, consider other formats like PIC 9 or PIC X.
10. Frequently Asked Questions
Q: What if my COMP-3 data represents a negative number?
A: The sign nibble in COMP-3 determines whether the number is positive or negative. You’ll need to handle this appropriately. For instance, if the sign nibble is X’F1’, it indicates a negative number. You’d then convert it accordingly.
Q: Can I use the INSPECT statement for conversion?
A: While you can technically use the INSPECT statement, it’s generally not the most straightforward way to convert COMP-3 data.
Q: Is there a built-in function for this conversion?
A: There’s no dedicated function for converting COMP-3 directly. You’ll need to use custom code like the example provided.
Q: Why is COMP-3 still used in modern applications?
A: While COMP-3 has its limitations in terms of readability, it’s still used in certain situations, particularly for legacy systems where space efficiency is a primary concern.
Q: How do I handle decimals in COMP-3 data?
A: Decimals are represented in COMP-3 by placing a sign bit in the rightmost nibble of the field. For example, to represent 12.34, you would use the following:
“`cobol
01 WS-COMP3-DATA PIC S9(4)V9(2) COMP-3 VALUE 12.34.
“`
You would then need to extract and manipulate the digits and decimal point separately.
Remember, mastering COMP-3 is a valuable skill for any COBOL programmer. Understanding its workings and conversion techniques can help you manage and interpret data effectively.
Converting comp-3 back to a human readable format
The best option is to do the conversion on the mainframe / Cobol and transfer a Text file. Alternatively There are projects like coboltocsv which will convert a Stack Overflow
Convert COMP-3 into readable format – IBM Mainframe Community
COBOL Programming: How do i display a comp-3 variable in readable format in COBOL. IBM Mainframe Community
COBOL COMP-3 | Packed-decimal
To define a COMP-3 variable in the COBOL program, we use the USAGE IS COMP-3 clause in the DATA DIVISION. For example – 01 WS-PDN PIC 9(5) USAGE IS COMP-3. Mainframestechhelp
COMP-3 in COBOL – GeeksforGeeks
For WS-VALC PIC S9(3) USAGE IS COMP-3, so here we can take it as (3+1)/2 which is equal to 2bytes size. Similarly, in the 3byte variable which is WS-VALD GeeksForGeeks
Convert packed decimal (comp-3) to readable format
I have an input file with Comp-3 data from column 113-117 with picture clause S9(10)V99. Total lenght of input file is 292 bytes. My requirement is to convert comp-3 mvsforums.com
Comp-3 fields Conversion – All other Mainframe Topics – IBM
I have a mainframe file which has 400+ columns are COMP-3 fields. Please let me know to convert all COMP-3 fields to readable format ( CSV or excel ). IBM Mainframe Forum
convert COMP-3 data in readable mode – MainframeWizard.com
One option is to read this file using another COBOL program and use MOVE for each variable to edited PICTURE variables, but I am looking for an alternative and easier mainframewizard.com
How to convert COMP-3 to Regular Number? – COBOL General
The comp-3 format means: x’12345c’ for +12345. x’12345d’ for -12345. x’12345f’ for 12345. so you can compare the valid hexadecimal values to find out what Tek-Tips Forums
Convert COMP to readable format – IBM Mainframe Community
DFSORT/ICETOOL: Hi, How to convert comp variable to readable format using SORT. i have a o/p file first 2 fields are in comp format. in… ibmmainframes.com
Converting Numeric Formats (Zd To Pd, Zd To Bi And Reverse)
Cobol: Zoned Decimal And Packed Decimal
Cobol Comp, Comp1, Comp2, Comp3 Variables
Cobol Lesson 120 – Comp-3 Packed Decimal Numerics
Lab 8 – Working With Comp-3 Using Cobol
Link to this article: how to convert comp 3 to readable format in cobol.
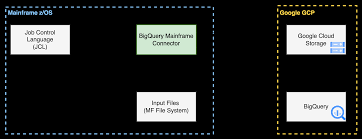
See more articles in the same category here: blog https://countrymusicstop.com/wiki