What data types are JSON serializable?
Let’s dive a bit deeper into why these data types work so well with JSON:
Booleans: JSON uses the keywords true and false to represent these values, making it straightforward to serialize boolean C++ types.
Integers: JSON represents integers with whole numbers, which aligns perfectly with C++’s integer types. Whether it’s a short, int, or long, it can be serialized directly into a JSON number.
Floating-Point Numbers: JSON uses the standard decimal representation for floating-point numbers, so C++ types like float and double translate smoothly into JSON numbers.
Strings: JSON employs strings for textual data, and both AZstd::string and OSString in C++ are used to represent strings. This direct correspondence makes serialization seamless.
JSON’s simplicity and the direct mapping of these common data types make it an efficient and reliable choice for data serialization.
What is not JSON serializable error in Python?
Let’s break down why this happens. When you use the `json.dumps()` function to serialize Python objects, it expects simple, fundamental data types like:
Strings
Numbers (integers and floats)
Booleans (True or False)
Lists
Dictionaries
But sometimes you’ll run into trouble if your object has custom attributes, methods, or references that don’t fit the JSON mold.
Here’s a quick example: Imagine you have a class called `Dog` representing your furry friend.
“`python
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
self.bark = “Woof!”
“`
You can create an instance of `Dog`:
“`python
my_dog = Dog(“Buddy”, “Golden Retriever”)
“`
Now, if you try to serialize `my_dog` to JSON:
“`python
import json
json.dumps(my_dog)
“`
You’ll get the infamous “not JSON serializable” error. This is because `my_dog` has the `bark` attribute, which is a function, and JSON can’t handle functions.
Here are the main culprits that can cause the “not JSON serializable” error:
Custom Objects: As we saw with the `Dog` class, any object with methods or non-serializable attributes will cause this error.
Functions: JSON doesn’t support functions, so trying to serialize a function directly will fail.
Sets: While Python sets are great for unique collections, they aren’t directly serializable to JSON.
Lambda Functions: These are anonymous functions, and just like regular functions, they can’t be serialized.
What can you do about it?
You have a few options to work around this error.
Convert the Object to a Dictionary: The most common approach is to convert your custom object to a dictionary. This involves creating a dictionary representation of your object, including its attributes.
“`python
def dog_to_dict(dog):
return {‘name’: dog.name, ‘breed’: dog.breed}
dog_dict = dog_to_dict(my_dog)
json_string = json.dumps(dog_dict)
“`
Use a Custom Encoder: You can create a custom JSON encoder class to handle your specific object types. This lets you control how objects are serialized. This is helpful if you want to customize how certain attributes are represented in your JSON.
Simplify the Object: Sometimes the simplest solution is to just remove the non-serializable elements from your object before attempting to serialize it.
Use a Serialization Library: If you’re dealing with complex objects, consider using a more robust serialization library like `pickle`. However, remember that `pickle` can be a security risk, so use it cautiously, especially when working with untrusted data.
By understanding what makes an object JSON serializable and the options for handling non-serializable elements, you can overcome this error and get your Python data into JSON format.
Is Python dictionary JSON serializable?
Let’s dive a bit deeper:
JSON (JavaScript Object Notation) is a lightweight data-interchange format that’s incredibly popular for exchanging data between applications. Think of it as a universal language for computers to talk to each other, and Python dictionaries fit right in!
Python’s `json` module provides functions like `json.dumps()` for converting a Python dictionary into a JSON string. This string is then easily saved to a file.
Here’s a simple example:
“`python
import json
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
json_string = json.dumps(my_dict)
print(json_string)
“`
Output:
“`
{“name”: “Alice”, “age”: 30, “city”: “New York”}
“`
To read JSON data from a file back into a Python dictionary, you use `json.loads()`.
What about other serialization methods? While Python dictionaries are readily JSON serializable, you might come across other methods like `pickle` or `shelve`. These methods are useful for saving more complex Python objects, but they are not directly compatible with JSON.
Key Takeaways:
* Python dictionaries are JSON serializable, making it easy to store and retrieve data in a standard format.
* The `json` module provides the tools for converting between Python dictionaries and JSON strings.
* While other methods like `pickle` exist, they are not compatible with JSON and are best reserved for specific scenarios.
Let me know if you have any other questions!
What is a serialized JSON object?
Think of it like taking a photo of your favorite object. You can then store the photo or send it to someone else. When you need the object back, you can just look at the photo and reconstruct it. Serialization works similarly, it captures the state of your object and stores it in a way that can be used to recreate the object later. Deserialization is the opposite process, where you take the stored snapshot and turn it back into a usable object.
JSON is a popular choice for storing and transmitting data because it’s a simple and human-readable format. It uses key-value pairs, which are similar to how we organize information in our everyday lives. For example, a person might be described using key-value pairs like “Name”: “John Doe”, “Age”: 30, “City”: “New York”. This structure makes it easy for both humans and computers to understand and work with the data.
When you serialize an object into JSON, you essentially convert its properties and values into this key-value pair format. This allows you to easily store or transmit the data. When you need to use the data again, you can deserialize it back into an object, bringing the data back to life.
What are the JSON scalar data types?
JSON values can be primitive or structured. Primitive values are called scalars and represent a single value. There are four scalar data types in JSON:
string: A string is a sequence of characters enclosed in double quotes. For example, `”Hello, world!”` is a string.
number: A number represents a numeric value. It can be an integer or a floating-point number. For example, `123` and `3.14159` are both numbers.
boolean: A boolean represents a truth value, which can be either `true` or `false`.
null: Null represents the absence of a value.
Let’s break down these scalar data types in more detail:
Strings
Strings are used to represent text. They are enclosed in double quotes. You can include special characters in strings by using escape sequences. For example, to represent a double quote within a string, you would use `\”`. Think of it like adding a special code to say “Hey, this quote is part of the text, not the end of the string!”
Numbers
Numbers in JSON can be integers or floating-point numbers. JSON doesn’t distinguish between different types of numbers, so `123` and `123.0` are both valid numbers.
Booleans
Booleans represent truth values. They can be either `true` or `false`. Think of these as simple on/off switches in your JSON data.
Null
Null represents the absence of a value. It’s like saying “Nothing is here.”
These scalar data types form the foundation of JSON and are used to build complex data structures. Understanding them is key to working with JSON effectively!
Can JSON encode bytes?
Let’s dive deeper into why you can’t directly encode bytes into JSON and how you can work around this limitation.
JSON, short for JavaScript Object Notation, is a human-readable format for data exchange. It uses text-based representations of data structures like lists, dictionaries, numbers, and strings. The core of JSON is built on the concept of text, making it inherently unsuitable for direct encoding of binary data like bytes. Think of it this way: if you try to shove a block of wood into a box meant for books, it won’t fit.
However, there are ways to bridge this gap. You can convert your bytes data into a suitable representation for JSON encoding. One approach is to base64-encode your bytes data. This process translates the binary data into a string that represents the original bytes in a printable, ASCII-safe format.
Here’s an example of how you could do this:
“`python
import json
import base64
# Your bytes data
my_bytes = b’This is some binary data’
# Base64 encode the bytes
encoded_bytes = base64.b64encode(my_bytes).decode(‘ascii’)
# Create a dictionary with the encoded data
data = {‘data’: encoded_bytes}
# Encode the dictionary as JSON
json_string = json.dumps(data)
# Write the JSON string to a file
with open(‘my_data.json’, ‘w’) as f:
f.write(json_string)
“`
When you decode the JSON later, you can then use the base64 module to convert the string back to its original bytes form.
Remember, although JSON itself doesn’t directly handle bytes, you can use encoding techniques like base64 to represent your binary data in a text-based form compatible with JSON. This allows you to effectively store and transfer your data using the convenient JSON format.
How do you convert an object type to JSON in Python?
Now, how does it work? Every Python object has a special attribute called __dict__. This attribute acts like a storage container for all the object’s properties, kind of like a dictionary. dumps() essentially looks at this __dict__ and uses its information to create the JSON representation.
Let me show you an example. Imagine you have a class called MyObject, with a couple of attributes like name and age.
“`python
class MyObject:
def __init__(self, name, age):
self.name = name
self.age = age
my_object = MyObject(“Alice”, 30)
import json
json_string = json.dumps(my_object.__dict__)
print(json_string)
“`
This code snippet first creates an instance of MyObject named my_object and initializes it with the name “Alice” and age 30. Then it uses the json.dumps() function to convert the my_object.__dict__ into a JSON string. The output would look like this: `{“name”: “Alice”, “age”: 30}`.
The beauty of this is that you can now easily use this JSON string to share data with other systems or applications that might be expecting data in this format.
How to create a JSON serializable object in Python?
The easiest way to achieve this is to leverage the __dict__ attribute that every Python object possesses. This attribute behaves like a dictionary, storing your object’s instance variables.
Here’s a breakdown of how you can use it:
“`python
import json
class MyObject:
def __init__(self, name, age):
self.name = name
self.age = age
my_object = MyObject(“Alice”, 30)
# Convert the object to JSON using the __dict__ attribute
json_data = json.dumps(my_object.__dict__)
print(json_data) # Output: {“name”: “Alice”, “age”: 30}
“`
The code demonstrates how to define a class MyObject with instance variables name and age. We create an instance of this class and then use `json.dumps` to convert it to JSON. The key here is `my_object.__dict__` which gives us a dictionary representation of the object’s attributes, allowing for easy serialization.
Understanding the Importance of __dict__
The __dict__ attribute plays a crucial role in enabling serialization. Let’s consider why:
Dictionary Format: JSON is fundamentally a data exchange format based on key-value pairs. Dictionaries in Python naturally align with this structure, making them perfect for conversion to JSON.
Object to Dictionary: The __dict__ attribute offers a seamless way to represent your object’s instance variables as a dictionary.
Simplifying Serialization: Using __dict__ eliminates the need for custom serialization logic, keeping your code cleaner and more efficient.
Remember: While this method is straightforward, you should be mindful of data types. If your object contains custom classes or complex data structures, you might need additional logic to ensure successful serialization. The __dict__ attribute provides a foundational building block for making your Python objects JSON-compatible.
See more here: How To Convert Bytes Object To Json In Python? | Object Of Type Bytes Is Not Json Serializable
What is type error if object of type bytes is not JSON serializable?
The reason this happens is because JSON (JavaScript Object Notation) works with strings, not raw bytes. Think of it like trying to put a puzzle piece into the wrong spot – the shapes don’t match!
To fix this, you need to decode the bytes object into a string first. The decode() method is your friend here! It lets you convert the bytes to a string, which JSON can then handle easily.
Here’s a quick example of how this works:
“`python
import json
my_bytes = b'{“name”: “Alice”, “age”: 30}’
# Decode the bytes to a string
my_string = my_bytes.decode(‘utf-8’)
# Now you can convert the string to JSON
my_json = json.loads(my_string)
print(my_json)
“`
In this example, `my_bytes` holds a bytes object that represents JSON data. By calling `my_bytes.decode(‘utf-8’)`, we convert it to a string, which is then successfully loaded as a JSON object using `json.loads()`.
Remember: Always use the correct encoding (like ‘utf-8’) when decoding bytes to ensure your data is properly translated into a readable string.
Let me explain this error in more detail. You see, the Python `json` module is designed to work with strings which are essentially sequences of characters. However, a bytes object is a sequence of raw bytes, which are the fundamental units of data in a computer.
Think of it this way: If you have a book written in a foreign language, you can’t understand it unless you know how to translate it into your own language. Similarly, the `json` module needs a string, a language it understands, to process the data. Trying to convert a bytes object directly to JSON is like trying to read that foreign language book without knowing any translations.
That’s why you encounter the TypeError: Object of type bytes is not JSON serializable error. It’s simply saying that the `json` module can’t understand the raw bytes and needs them to be translated into a string first.
The decode() method is like your translator for the `json` module. It takes the bytes object and translates it into a string using a specific encoding like ‘utf-8’. This allows the `json` module to understand the data and process it accordingly.
How to serialize complex Python objects into JSON?
jsonpickle is a fantastic tool for serializing complex Python objects into JSON and back again. Think of it as a bridge between your Python world and the JSON format. It handles the conversion of complex objects into a JSON representation that can be easily stored or transmitted, then seamlessly reconstructs those objects when you need them back in Python.
So how does jsonpickle work its magic? It uses a clever technique called object graph traversal. Essentially, it walks through your object, identifying its attributes, and converting them into a JSON-compatible format. This includes handling relationships between objects, like when one object contains references to other objects.
Let’s look at an example:
“`python
import jsonpickle
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person = Person(“Alice”, 30)
# Serialize the Person object to JSON
json_string = jsonpickle.encode(person)
print(json_string)
# Deserialize the JSON back into a Person object
person_from_json = jsonpickle.decode(json_string)
print(person_from_json.name) # Output: Alice
“`
In this example, we define a simple `Person` class and create an instance. `jsonpickle.encode()` converts this object into a JSON string, and `jsonpickle.decode()` brings it back to life as a `Person` object. This makes it possible to store or share your complex Python objects in a way that can be easily understood and used by other systems or applications.
Let me know if you want to explore more advanced scenarios or dive deeper into the nuts and bolts of jsonpickle. I’m always ready to help you navigate the world of Python serialization!
How to solve serialize a bytes object to a JSON string?
“`python
import json
# Let’s say you have a bytes object
my_bytes = b'{“name”: “Alice”, “age”: 30}’
# Decode the bytes object to a string
my_string = my_bytes.decode(‘utf-8’) # Assuming UTF-8 encoding
# Now you can safely serialize the string to JSON
json_string = json.dumps(my_string)
print(json_string) # Output: ‘{“name”: “Alice”, “age”: 30}’
“`
In the example, we first create a bytes object containing a JSON structure. We then use .decode(‘utf-8’) to convert it into a string assuming it was encoded in UTF-8. Once we have the string, we can use json.dumps() to serialize it into a valid JSON string.
Let’s dive a bit deeper into why this is necessary. JSON (JavaScript Object Notation) is a human-readable data format that is commonly used for exchanging data between applications and services. It works with strings, numbers, booleans, lists, and dictionaries, but not directly with binary data like bytes objects.
When you try to serialize a bytes object to JSON, Python doesn’t know how to represent the raw binary data as a JSON structure. By converting the bytes object to a string using .decode(), you tell Python to interpret the binary data as characters, making it compatible with the JSON format.
Remember to always specify the correct encoding when decoding your bytes object. The most common encoding is UTF-8, which is widely used for representing text in various languages.
Why can’t I serialize a JSON object?
Think of it like this: JSON is like a language that only understands words (strings). Bytes are like raw sounds that need to be translated into words before they can be understood.
Here’s how you can decode the binary data into a Python string:
“`python
import json
binary_data = b'{“key”: “value”}’
decoded_string = binary_data.decode(‘utf-8’) # Assuming UTF-8 encoding
json_data = json.loads(decoded_string)
print(json_data)
“`
In this example, we first decode the bytes object (`binary_data`) into a string using the `decode()` method. We assume UTF-8 encoding, which is a common standard for text. After that, we can use `json.loads()` to parse the string into a Python dictionary.
Understanding Encoding
Encoding is the process of converting data from one format to another. In this case, we’re converting from bytes (binary data) to strings (text). There are different encoding schemes available, such as UTF-8, ASCII, and Latin-1. The correct encoding depends on the type of data you’re working with.
Important Note: Make sure you choose the correct encoding that matches the way your binary data was originally encoded. Using the wrong encoding can lead to incorrect data or even errors.
By decoding the bytes into a string, you can then serialize it into JSON using `json.dumps()`. This will give you the JSON representation of your data, which you can then use for tasks like sending data over the network or saving it to a file.
See more new information: countrymusicstop.com
Object Of Type Bytes Is Not Json Serializable: Solving The Python Error
JSON’s Rules
First, let’s talk about JSON. JSON stands for JavaScript Object Notation. It’s like a universal language for exchanging data between different applications and systems. Imagine it as a way to pack information into a neat, organized box that everyone can understand.
Here’s the thing: JSON only allows certain data types, like strings, numbers, booleans, lists, and dictionaries.
Think of it this way: JSON is a picky eater. It likes its data to be in specific forms. It’s like trying to put a puzzle piece that doesn’t fit. Bytes are like those oddly shaped puzzle pieces.
Bytes: A Bit Different
Bytes in Python are sequences of raw bytes. They’re like a bunch of little boxes holding numbers representing data. They’re not inherently human-readable, and they don’t follow the same rules as the data types that JSON allows.
You’re essentially trying to stuff a square peg into a round hole. You need to translate those bytes into a form that JSON understands.
The Solution: Conversion
Alright, let’s fix this. The trick is to convert the bytes into a JSON-friendly format, like a string. There are two main ways to do this:
Base64 Encoding: Think of it like a special code that turns your bytes into a string of characters. It’s a common way to represent binary data in text formats.
Hexadecimal Encoding: This is another way to represent bytes in a string format. It uses hexadecimal numbers, which use a system of 16 digits.
Hands-On Example: Base64 Encoding
Let’s see this in action. Here’s how you can encode bytes using Base64 in Python:
“`python
import base64
my_bytes = b’This is some data’
# Convert bytes to Base64-encoded string
encoded_string = base64.b64encode(my_bytes).decode(‘utf-8’)
# Now you can use ‘encoded_string’ in your JSON
print(encoded_string)
“`
This code will output: ‘VGhpcyBpcyBzb21lIGRhdGE=’
Decoding: Getting the Bytes Back
You can also decode the Base64-encoded string back into bytes when you need to use it again.
“`python
import base64
encoded_string = ‘VGhpcyBpcyBzb21lIGRhdGE=’
# Decode the Base64-encoded string
decoded_bytes = base64.b64decode(encoded_string)
print(decoded_bytes)
“`
This will output: b’This is some data’
JSON Serialization and Deserialization
Now you’re probably wondering how to incorporate this into your JSON serialization process.
Here’s a quick example:
“`python
import json
import base64
data = {
‘name’: ‘Alice’,
‘image’: b’This is some image data’
}
# Encode the bytes to Base64
data[‘image’] = base64.b64encode(data[‘image’]).decode(‘utf-8’)
# Convert the dictionary to JSON
json_string = json.dumps(data)
print(json_string)
“`
FAQ
#1. What is JSON Serialization?
JSON serialization is the process of converting a Python object (like a dictionary or list) into a JSON string. Think of it like packing your data into a neat box that can be sent over the internet.
#2. Why Can’t I Directly Serialize Bytes?
JSON doesn’t have a built-in way to represent bytes directly. It works with data types like strings, numbers, and lists.
#3. How Do I Decode Base64-Encoded Data?
You can use the `base64.b64decode()` function to decode Base64-encoded data back into bytes.
#4. What are Other Ways to Encode Bytes?
You can also use other encoding schemes, such as Hexadecimal or UTF-8. Choose the one that works best for your situation.
#5. What if I’m Dealing with Files?
If you have a file that you want to include in your JSON data, you can read it as bytes, encode it, and then include it in your JSON.
Remember, the key to working with bytes and JSON is to understand that they speak different languages. By using encoding and decoding, you can bridge the gap and make sure your data is understood by both.
How to deal with TypeError: Object of type ‘bytes’ is not JSON …
A user asks how to convert a base64-encoded image to JSON format without getting the error “Object of type ‘bytes’ is not JSON serializable”. Three answers suggest Stack Overflow
TypeError: Object of type ‘bytes’ is not JSON serializable
A user asks why they get a TypeError: Object of type ‘bytes’ is not JSON serializable when using scrapy to create a bot. Several answers explain the cause and solution of the Stack Overflow
How to Solve Python TypeError: Object of type bytes is not JSON …
This error occurs when you try to serialize a bytes object to a JSON string using the json.dumps() method. You can solve this error by decoding the bytes object to a string The Research Scientist Pod
Make a Python Class JSON Serializable – PYnative
Learn how to encode and decode custom Python objects into JSON format using different methods. See examples of subclassing JSONEncoder, implementing toJSON() method, using jsonpickle PYnative
TypeError: Object of type function is not JSON serializable
Learn how to fix the error when trying to serialize a function to JSON in Python. The solution is to call the function and serialize its return value, not the function bobbyhadz
Object of type is not JSON serializable in Python –
Learn why “Object of type is not JSON serializable” happens and how to solve it with different methods. See examples of encoding bytes, writing custom JSON encoder, and using jsonpickle library. LinuxPip
5 Best Ways to Serialize Bytes to JSON in Python
Standard JSON serializers raise an error when they encounter byte-like objects because JSON is a text-based format. This article provides practical methods to Finxter
Handling JSON Serialization Error in Python 3: Object of type ‘bytes’
Learn how to deal with the TypeError: Object of type ‘bytes’ is not JSON serializable when working with JSON data in Python. See examples of decoding bytes objects to strings dnmtechs.com
How to fix TypeError: Object of type function is not JSON serializable …
TypeError: Object of type function is not JSON serializable. The error occurs because the JSON encoder that’s used in the dumps() method doesn’t support sebhastian.com
Object Of Type Bytes Is Not Json Serializable
Object Of Type X Is Not Json Serializable – Object Serialization In Python Part 1
Python Flask Tutorial #40 Typeerror: Object Of Type Response Is Not Json Serializable
Python Object Of Type Bytes Is Not Json Serializable
How To Fix Typeerror: Object Of Type ‘Set’ Is Not Json Serializable When Try… In Python
Link to this article: object of type bytes is not json serializable.
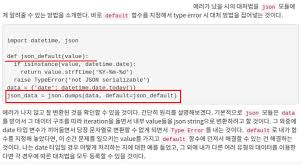
See more articles in the same category here: blog https://countrymusicstop.com/wiki